Coding Guru
JAVA.LANG PACKAGE
JAVA.LANG:
-
The most commonly required class and interfaces which are required for writing any java program whether it is simple or complex, are encapsulated into separate package which is nothing but the lang package.
-
It is not required to import lang package explicitly because by default it is available to every java program.
-
The following are some of the commonly used classes in lang package.
-
Object
-
String
-
String Buffer
-
String Builder
-
Wrapper Classes
1Object:
The most common methods which are required for any java object which are encapsulated into separate class which is nothing but object class.Sun people made this class as parent for all java classes so that its methods are bydefault available to every java class automatically.
Every class in java is the child class of object either directly or indirectly, if our class won’t extend any other class than any other class is direct child class of object.
Ex:
Class A Object A
{-------
-------- }
If our class extends any other class then our class is not direct child class of object,it extends object class indirectly.
Ex:
Class A extends B Object
{---------------------- B
-----------------------}
A
The object class defines the following 11 methods:
1.1Public String toString ()
1.2Protected native Object clone () throws CloneNotSupportException
1.3Public final class getClass();
1.4Protected void Finalize () throws Throwable
1.5Public final void wait () throws interrupted Exception
1.6Public final native void wait (long ms) throws IE
1.7Public final void wait (long ms) throws IE
1.8Public final native void notify ();
1.9Public final native void notifyAll ();
1.1toString () method:
-
We can use this method to find String Representation of the Object.
-
Whenever we are trying to print any object reference internally toString () method will be executed.
-
Ex:
Class Student
{
String name;
Int rollno;
Student (String name, int rollno) {
This.name=name;
This. Rollno=rollno;
}
P.s.v.m (String args []) {
Student s1= new Student (“durga”, 101);
Student s2= new Student (“srinu”, 102);
S.o.pln (s1); èS.o.pln (s1.toString ()); Student@3e2845
S.o.pln (s2); èS.o.pln (s2.toString ()); Student@19821f
}
}
-
In the above case Object class toString () method get executed which is implemented as follows.
-
Public String toString ()
{
Return getClass ().get Name+ “@”+Integer.toHexString (hashCode ());
}
classname@hexadecimal String Representation of hashcode.
-
To provide our own String Representation we have to override toString () in our class which is highly Recommended.
-
Whenever we are trying to print Student Object reference to return his name and roll number we have to override toString() as follows.
-
If we are giving opportunity to our class toString () method then it may not call hashcode() method.
Public String toString () {
Return name;
Return name+ “----“+rollno;
Return “this is Student with name: “+name+”, withrollno:” +rollno;
}
In string, String Buffer and in a wrapper classes toString () method is overridden to return proper string form. Hence, it is highly recommended to override toString () method in our class also.
Ex:
Class Test {
Public String toString () {
Return “test”;
}
P.s.v.m (String args []) {
Test t = new Test ();
String s =new String (“Durga”);
Integer I =new Integer (10);
S.o.pln (t); test test@a23504
S.o.pln (s); Durga
S.o.pln (i); 10
}
}
1.10Public native int hashCode ()
For Every object JVM will assign one unique id which is nothing but hashcode.
JVM uses hashcode which will save objects into the hashtable or hashset or hashmap
Based on our Requirement we can generate hashcode by overriding hashcode () method in our class.
If we are not overriding hashcode () method then object class. Hashcode () method will be executed which generates hashcode based on the Address of the object but whenever we are overriding hashcode () method then hashcode is no longer related to the address of the Object.
Overriding hashcode () method is said to be proper if for every object we have to generate a unique number.
Ex:
Case1: case2:
Class Student { Class Student {
------------------ -------------------
------------------- --------------------
Public int hashcode () { Public int hashcode () {
Return 100; return “rollno”;
} }
} }
Case 1:
It is improper way of overriding hashcode () because we are generating some hashcode for every object.
Case 2:
It is the proper way of overriding hashcode () because we are generating a different hashcode for every object.
1.11toString () Vs hashcode ():
Ex:
Class Test { class Test {
Int I; Int I;
Test (Int I) { Test (Int I) {
This.i =I; this.i=I;
} }
P.s.v.m (String args []) { Public Int hashCode () {
Return I ;}
Test t1 =new Test (10); P.s.v.m (String args []) {
Test t2 = new Test (100); Test t1 =new Test (10);
S.o.pln (t1); Test@1a3b2b Test t2 =new Test (100);
S.o.pln (t2); Test@2a4b3a S.o.pln (t1); Test@a
} S.o.pln (t2); Test@64
} }
}
Object toString () Object toString ()
Object hashcode () Test hashCode
Class Test {
Int I;
Test (Int i) {
this.i=I;
} Public Int hashCode () {
Return I;}
P.s.v.m (String args []) {
Test t1 =new Test (10);
Test t2 =new Test (100);
S.o.pln (t1); Test@a
S.o.pln (t2); Test@64
}
}
Test toString ()
If we are giving opportunity to object class toString () method then it will call internally hash code () method.
-
1.12Public Boolean equals (Object o)
-
We can use equals () method to check equality of two objects.
Public Boolean equals(Object o)
Ex:
Class student {
String name;
Int rollno;
Student (String name, Int rollno) {
This.name=name;
This.rollno = rollno;
}
P.s.v.m (String args [] ) {
Student s1 =new Student (“durga” ,101);
Student s2 =new Student (“pavan” ,102);
Student s3 =new Student (“durga” ,101);
Student s4 =s1;
S.o.pln (s1.equals (s2)); //false
S.o.pln (s1.equals (s3)); //false
S.o.pln (s1.equals (s4)); //true
}
}
In the above case object class equals () method will be executed which is always meant for reference comparison (address comparison).
If two reference pointing to the same object then only equals () method returns true. This behavior is exactly same as ==operator.
If we want to perform content comparison instead of reference comparison we have to override equals () method in our class.
Whenever, we are overriding equals () method we have to consider the following things:
1.12.1What is the meaning of equality
1.12.2In the case of difference type of objects equals method should return falsebut not classcastException.
1.12.3If we are trying to pass null argument our equals method should returns false but not a NullPointerException.
The Following is the valid way of overriding equals() method in Student class.
Ex:
Public Boolean equals (object o) {
Try {
String name1=this.name;
Int rollno1 = this.rollno;
Student s2= (Student) o;
Student name2 =s2.name;
Int rollno2 = s2.rollno;
If(name1.equals(name2) && rollno1 == rollno2) {
Return true;
}
Else {
Return false;
}
}
Catch(CCE e) {
Return false;
}
Catch(NPE e) {
Return false;
}
Student s1 =new Student (“durga”,101);
Student s2 =new Student (“pavan”,102);
Student s3 =new Student (“durga”,101);
Student s4 =s1;
S.o.pln (s1.equals (s2)); ///false
S.o.pln (s1.equals (s3));///true
S.o.pln (s1.equals (s4));///true
S.o.pln (s1.equals (“durga”));///false
}
}
1.13 Short way of writing equals() method:
Public Boolean equals (object o) {
Try
{
Student s2= (student) o;
If (name. equals (s2.name) && rollno ==s2.rollno) {
Return true;
Else
Return false;
Catch(CCE e) {
Return false;
}
Catch(CCE e) {
Return false;
}
}
}
1.14 Relationship between == operator and equals() method:
If r1==r2 is true then r1.equals (r2) is always true.
If r1==r2 is false then r1.equals (r2) is always can’t be expected.
If r1.equals (r2)is true then r1==r2 is always can’t be expected
If r1.equals (r2)is false then r1==r2 is always false.
-
1.15 Difference between == operator and equals() method:
-
Note:
What is the difference between double equal operator (==) and .equals ()?
Ans: Double equal (==) operator is always meant for reference comparison, whereas .equals () method meant for content comparison.
Ex: String S1=new String (“durga”);
String S2=new String (“durga”);
S.o.pln (S1==S2); //false
S.o.pln (S1.equals (S2)); //true
àIn string classes .equals () is overridden for content comparison.
à In string Buffer class .equals () is not overridden for content comparison hence object class .equals () got executed which is meant for reference comparison.
àIn wrapper class .equals () is overridden for content comparison.
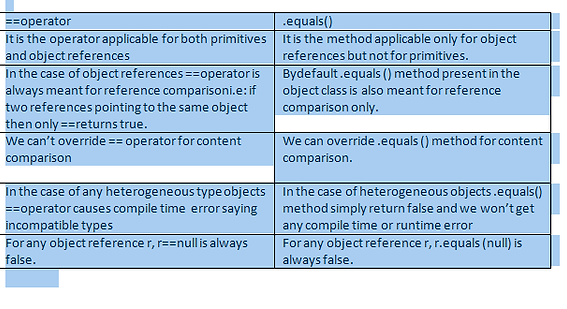